IEX Cloud Shutting Down: A Complete Python Migration Guide
- Nikhil Adithyan
- 12 minutes ago
- 9 min read
How to migrate from IEX Cloud to Alpha Vantage for stock quotes, historical prices, and financial statements using Python
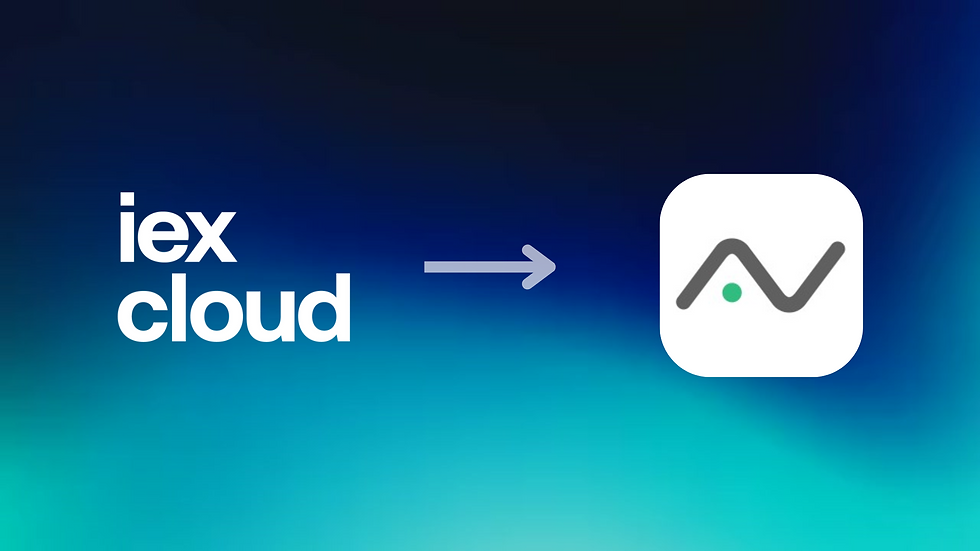
If you’ve used IEX Cloud for stock quotes, historical prices, or financials, you’ve probably already seen the news: IEX Cloud shut down on August 31, 2024. After that, all services went offline. No more quote, chart, or income endpoints. Everything’s gone.
For a lot of developers, this was a big deal. IEX Cloud was clean, developer-friendly, and easy to plug into projects. Whether you were building dashboards, backtests, or market data tools, it just worked.
But now that it’s shut down, the question is simple: what’s next?
In this article, I’ll walk through how to migrate your IEX Cloud workflows over to Alpha Vantage. If you’ve used endpoints like quote, chart, income, balance-sheet, or cash-flow, I’ll show you the equivalent APIs in Alpha Vantage and how to work with them.
No fluff. No sales talk. Just a guide that helps you keep your stuff running.
Let’s start with the basics, migrating your quote data.
1. Migrating Stock Quotes
The most common IEX Cloud endpoint by far was quote. It gave you the current price, volume, open, high, low, and daily change. Basically, everything you needed to display a live snapshot of a stock.
If you used it for dashboards, watchlists, or even lightweight trading tools, chances are your code depended on it heavily.
The good news is that Alpha Vantage offers a direct replacement. It’s called the Quote endpoint, and it works using the GLOBAL_QUOTE function.
Here’s a simple example:
And here’s what the response looks like:
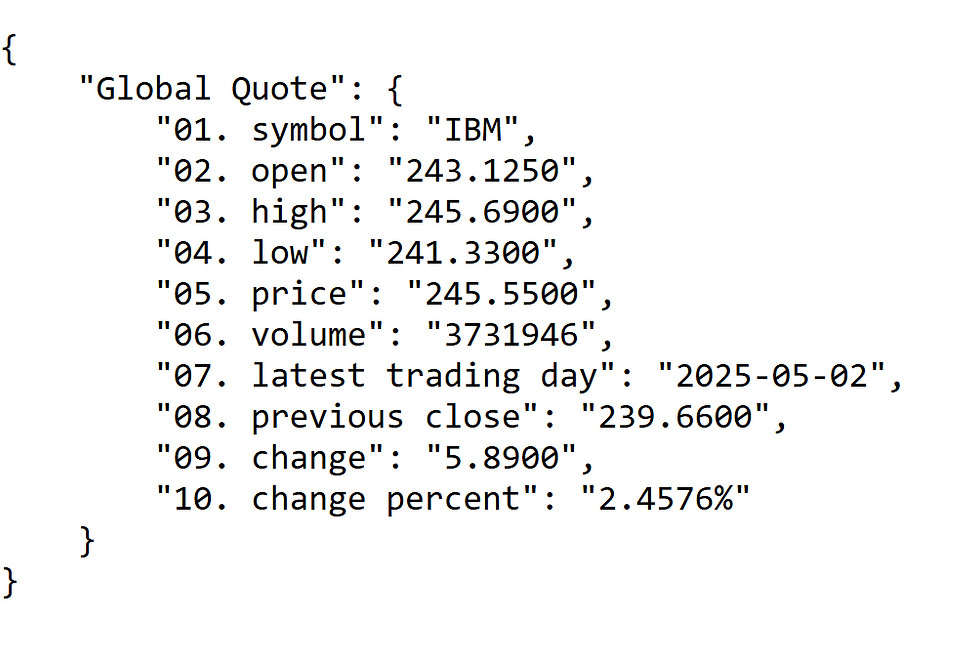
The field names are a bit verbose, each one starts with a number, but the data itself is clear. You get the current price, volume, percent change, previous close, and so on.
Here’s a quick Python example to pull and print the quote:
import requests
symbol = 'IBM'
api_key = 'YOUR_API_KEY'
url = f'https://www.alphavantage.co/query?function=GLOBAL_QUOTE&symbol={symbol}&apikey={api_key}'
response = requests.get(url).json()
quote = response['Global Quote']
print('Symbol:', quote['01. symbol'])
print('Price:', quote['05. price'])
print('Volume:', quote['06. volume'])
print('Change:', quote['09. change'], f"({quote['10. change percent']})")
This gives you the same core data that IEX Cloud's quote endpoint used to return.
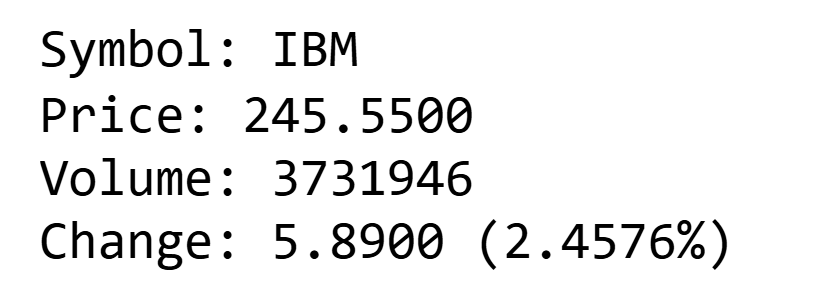
One thing to keep in mind is that, by default, Alpha Vantage updates this data at the end of each trading day. It’s not real-time unless you’re on a premium plan. If you just need daily snapshots or are running overnight processes, the free version works fine. But if your use case depends on real-time or delayed data for U.S. stocks, you’ll need to upgrade.
Also, be aware of rate limits if you’re calling this in a loop. Free users get 5 API calls per minute and 500 per day, so it helps to batch or cache requests if you're tracking multiple tickers.
Bottom line: if you were using IEX Cloud’s quote endpoint, Alpha Vantage’s Quote endpoint is a solid replacement. Just watch out for the field names and update cycle.
2. Migrating Historical Prices
If you were using IEX Cloud’s chart endpoint, chances are you were pulling historical prices for backtests, charting, or just basic time series analysis. It supported flexible ranges, things like 1d, 5d, 6m, max. You could get adjusted or unadjusted prices, depending on what you needed.
That endpoint is gone now. But the good news is, Alpha Vantage offers an equivalent that covers most of the same ground, the TIME_SERIES_DAILY_ADJUSTED endpoint.
Here’s a basic example of how it works:
https://www.alphavantage.co/query?function=TIME_SERIES_DAILY_ADJUSTED&symbol=IBM&apikey=YOUR_API_KEY
This returns daily historical data with:
Open, high, low, close
Adjusted close (post-split, post-dividend)
Volume
Dividend amount
Split coefficient
By default, you’ll get the last 100 days. If you want full history, you can add outputsize=full to the URL.
Here’s what a small slice of the response looks like:
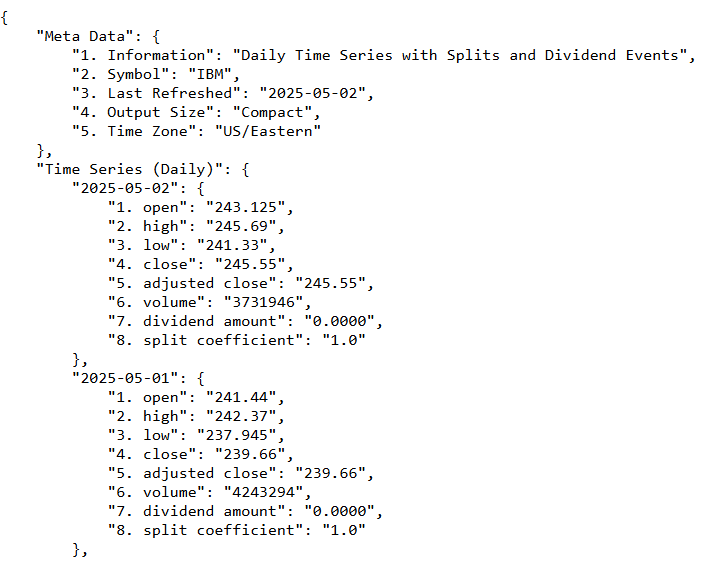
Each date is a key. Inside that, you get all the price fields as nested values. It’s different from IEX Cloud, which returned a list of objects. Here, you’ll need to loop through the keys or flatten it into a DataFrame.
Here’s a quick Python example to do that:
import requests
import pandas as pd
symbol = 'RELIANCE.BSE'
api_key = 'YOUR_API_KEY'
url = f'https://www.alphavantage.co/query?function=TIME_SERIES_DAILY_ADJUSTED&symbol={symbol}&outputsize=full&apikey={api_key}'
response = requests.get(url).json()
data = response['Time Series (Daily)']
df = pd.DataFrame(data).T
df = df.rename(columns={
"1. open": "open",
"2. high": "high",
"3. low": "low",
"4. close": "close",
"5. adjusted close": "adjusted_close",
"6. volume": "volume"
})
for col in df.columns:
df[col] = pd.to_numeric(df[col])
df.head()
This gives you a nice clean DataFrame with proper date indexing and float-typed columns, ready for charts, backtests, or whatever analysis you were running before.
A few things to keep in mind:
This endpoint only gives daily prices. If you were using IEX’s intraday history, you’ll need a different Alpha Vantage endpoint for that (like TIME_SERIES_INTRADAY).
You get adjusted close by default, which is great if you care about dividend- and split-adjusted pricing. No extra logic needed.
Alpha Vantage supports global tickers too, not just US stocks. You can query NSE, BSE, LSE, XETRA, TSX, and even China’s SSE/SHZ. The symbol format varies by exchange, but their docs have solid examples.
If you were relying on IEX Cloud’s chart endpoint for daily historical data, this one gets you 90% of the way there. The rest is just adapting your parsing logic.
3. Migrating Financial Statements
If you were using IEX Cloud’s fundamental endpoints to pull income statements, balance sheets, or cash flow data, you probably had a pipeline set up around them, maybe for valuation models, fundamental screeners, or quarterly report tracking.
Now that those endpoints are gone, you’ll need alternatives that give you the same level of access to structured financials.
Alpha Vantage has separate endpoints for each of the three core statements: income, balance sheet, and cash flow, and they all follow a similar format. Each one returns both annual and quarterly data, and most of the fields you’d expect are available right out of the box.
Let me start with the income statement.
Income Statement
The income endpoint on IEX Cloud gave you access to revenue, gross profit, net income, and other key profitability metrics, broken down by fiscal period. It was often used to calculate margins, growth rates, or to power earnings-based valuation models.
The Alpha Vantage equivalent is the INCOME_STATEMENT endpoint.
Here’s a basic example call:
The response includes both annualReports and quarterlyReports. Each one is a list of dictionaries, where each dictionary represents one fiscal period and contains fields like:
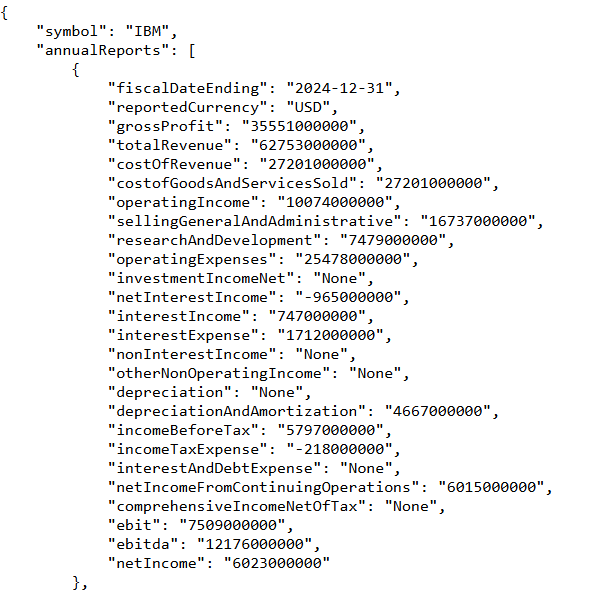
Here’s a quick example in Python to fetch and display the latest annual report:
import requests
import pandas as pd
symbol = 'IBM'
api_key = 'YOUR_API_KEY'
url = f'https://www.alphavantage.co/query?function=INCOME_STATEMENT&symbol={symbol}&apikey={api_key}'
response = requests.get(url).json()
annual_reports = response['annualReports']
df = pd.DataFrame(annual_reports)
cols = ['fiscalDateEnding', 'totalRevenue', 'grossProfit', 'operatingIncome', 'netIncome', 'ebitda']
df[cols].head()
This gives you clean, structured financials ready to plug into models or display in your app.
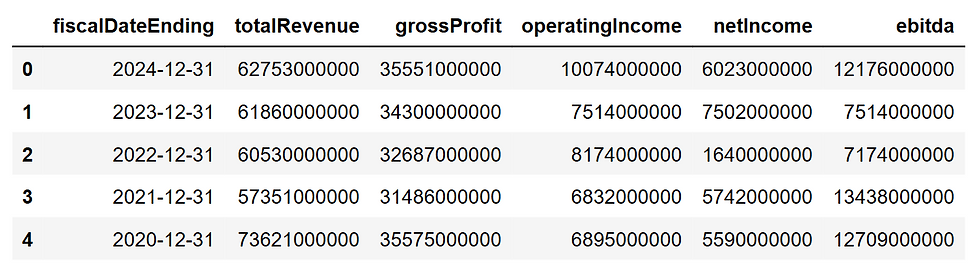
A couple of things to note:
Values are returned as strings, so you’ll need to convert them to int or float before doing any math.
Alpha Vantage includes both EBIT and EBITDA, which IEX Cloud didn’t always offer out of the box.
The endpoint gives you full historical depth, not just the latest year or quarter.
If you were using IEX’s income endpoint for any kind of profitability or growth analysis, this is a straight swap. The structure’s different, but the data’s all there.
Balance Sheet
IEX Cloud’s balance sheet endpoint was usually where you'd pull data on assets, liabilities, and equity. It helped with calculating debt ratios, working capital, book value, and other health checks on a company’s financial position.
Alpha Vantage’s equivalent is the BALANCE_SHEET endpoint. It gives you full-year and quarterly balance sheets in a clean JSON format, with breakdowns for current and non-current assets, liabilities, and total shareholder equity.
Here’s how a basic API call looks:
The response has two sections: annualReports and quarterlyReports. Each one gives you detailed numbers for everything from cash to long-term debt to retained earnings. Here's a trimmed example:
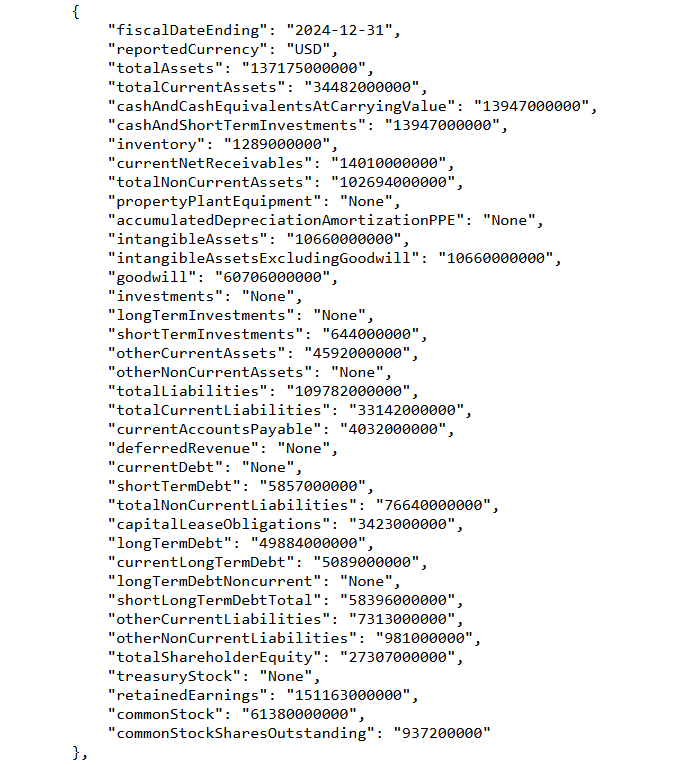
Here’s a quick way to fetch and view the annual balance sheet in Python:
import requests
import pandas as pd
symbol = 'IBM'
api_key = 'YOUR_API_KEY'
url = f'https://www.alphavantage.co/query?function=BALANCE_SHEET&symbol={symbol}&apikey={api_key}'
response = requests.get(url).json()
annual_reports = response['annualReports']
df = pd.DataFrame(annual_reports)
cols = ['fiscalDateEnding', 'totalAssets', 'totalLiabilities', 'totalShareholderEquity', 'longTermDebt']
df[cols].head()
Like the income statement, values are returned as strings. So you’ll need to convert them if you’re doing math.
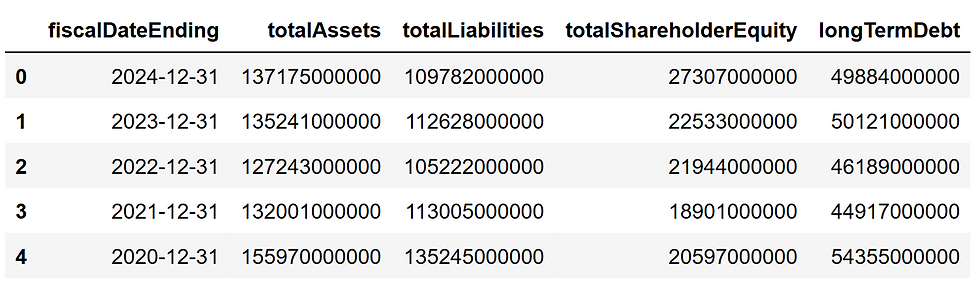
A few extra notes:
Field names are long and verbose, but pretty self-explanatory.
All numbers are reported in the company's native currency, check the reportedCurrency field if you're mixing tickers.
You get deep history, not just the latest quarter or year.
If you were using IEX Cloud’s balance sheet endpoint for any kind of solvency or asset analysis, this one’s an easy replacement. The only real change is adapting to the nested structure.
Cash Flow
If you’ve ever built a DCF model or just wanted to track how much real money a business was generating, you were probably using IEX Cloud’s cash flow endpoint. It gave you net cash from operating, investing, and financing activities, which are the real heartbeat of a company.
Here’s the basic call:
Just like the others, it returns both annualReports and quarterlyReports. Each report includes fields like:
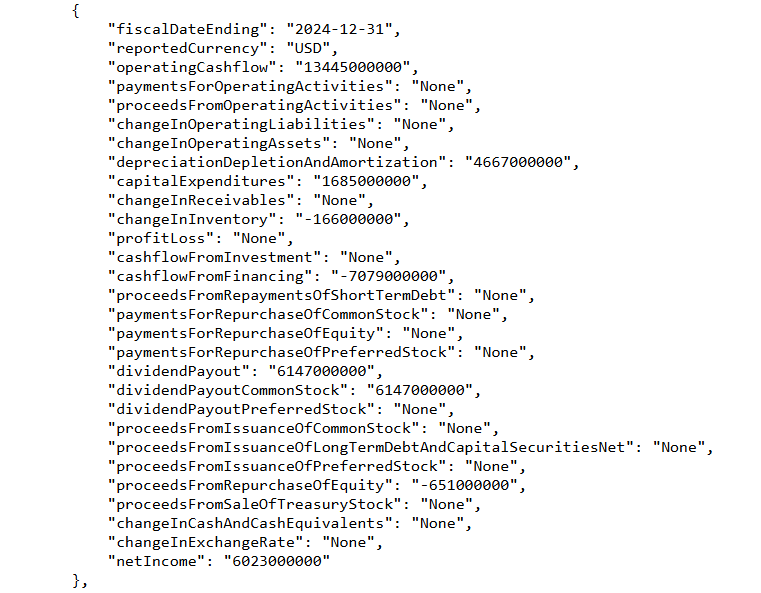
Here’s a quick example in Python to parse and work with the data:
import requests
import pandas as pd
symbol = 'IBM'
api_key = 'YOUR_API_KEY'
url = f'https://www.alphavantage.co/query?function=CASH_FLOW&symbol={symbol}&apikey={api_key}'
response = requests.get(url).json()
annual_reports = response['annualReports']
df = pd.DataFrame(annual_reports)
cols = ['fiscalDateEnding', 'operatingCashflow', 'capitalExpenditures', 'cashflowFromFinancing', 'dividendPayout']
df[cols].head()
Like with the other endpoints, you’ll need to cast the string values to numbers before doing any math. But everything is already organized in a way that makes it easy to plug into your existing pipeline.
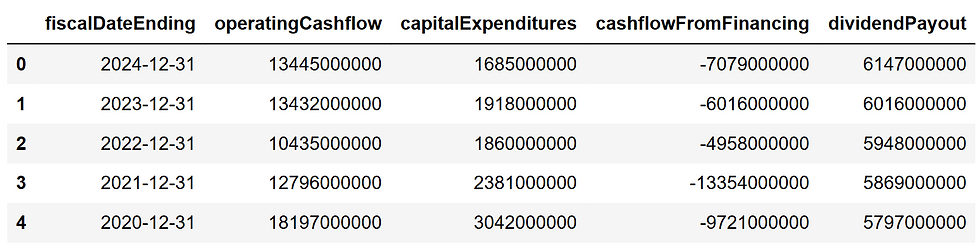
A couple of useful notes:
operatingCashflow is already post-working-capital, no need to manually adjust.
If you’re comparing to net income, both are reported in the same fiscal frame.
You can track trends in dividends, buybacks, and debt issuance cleanly across years.
So if IEX Cloud’s cash flow endpoint was powering any of your valuation models, payout ratio checks, or leverage tracking, this will cover it without needing much rework.
Migration Tips
Switching APIs always comes with a bit of friction. The data might be the same, but the formats, limits, and quirks are almost never a one-to-one match. If you’re moving from IEX Cloud to Alpha Vantage, here are a few things to keep in mind to make that process smoother.
a. Rate limits are real
Alpha Vantage’s free tier gives you 5 API calls per minute and 500 per day. If you're looping through dozens of tickers or running a daily cron job, you’ll hit those limits pretty quickly.
Best workaround? Add a short delay between requests (time.sleep(12) works fine for the minute cap) or cache responses locally so you’re not calling the same endpoint repeatedly. If you're building a dashboard or production app, it’s worth checking out their premium plans. It unlocks real-time data and higher throughput.
b. Expect to reshape the data
If you’re used to IEX’s flat JSON responses, Alpha Vantage will feel more nested. Historical endpoints (like TIME_SERIES_DAILY_ADJUSTED) use dates as keys, so you’ll need to .T (transpose) the DataFrame. Same with financials, every endpoint wraps values in long key names like "1. open", "5. adjusted close", and so on.
It’s nothing too complex, but it does mean rewriting some of your data processing logic.
c. Don’t migrate everything at once
Pick a couple of tickers, maybe ones you're actively tracking, and test your logic with those first. Make sure the values match your expectations, fields aren’t missing, and everything converts properly.
Once the code is stable, scaling to 50 or 500 tickers is just a matter of time management and batching.
d. Convert your data types early
Every value comes in as a string, even numbers. That means if you're doing math (like calculating margins, price changes, or ratios), you'll need to use pd.to_numeric() or float() early in your pipeline. Otherwise, you'll end up with some really weird results.
e. Use outputsize=compact by default
For historical endpoints, compact gives you the last 100 data points, which is usually enough for most use cases. If you're building a long-term model or pulling entire price histories, switch to outputsize=full. Just be ready for a larger payload and slower response time.
f. CSV is your friend
Every endpoint supports datatype=csv as a parameter. This lets you download the data as a file directly, which is useful for debugging, sharing with a team, or just inspecting raw values in Excel without writing any code.
g. Ensure the migration works both locally and on the cloud
If you are hosting your algorithmic trading algorithms on the cloud (e.g., Azure Linux VM, AWS Lambda, etc.), extra steps may be needed to “cloudify” your migration script. You may also want to enable autoscaling for the cloud services so that your business logic can scale with the intensity of usage.
Conclusion
If IEX Cloud was part of your workflow, the shutdown probably forced you to rethink a few things. But the good news is, you don’t have to start from scratch. Most of what you were doing, quotes, prices, and financials, can be rebuilt using Alpha Vantage with just a few changes in logic and formatting.
It’s not a perfect one-to-one swap, and some rewiring is needed. But once you adjust to the structure and limits, the coverage is solid, and the docs are clear.
I’ve personally found Alpha Vantage to be one of the more reliable options out there, especially if you're working on side projects, dashboards, or any Python-based tools. It just works.
Hope this guide helped you get your codebase back up and running.
Comments