Algorithmic Trading with Aroon Indicator in Python
- Nikhil Adithyan
- May 19, 2021
- 15 min read
Learn to detect market trends and make trades accordingly with a powerful indicator in python

There are a lot of technical indicators out there to be used for research or trading purposes but one common similarity among them is that they all serve only one specific task. For example, RSI can be used to identify overbought and oversold levels in a market, Choppiness Index can be used to observe the market volatility, and so on. But, today we are going to explore an indicator that can be used for either acknowledging the market trend or market volatility. Behold, the Aroon Indicator! In this article, we will discuss what the Aroon Indicator is all about, its usage and calculation, and how a trading strategy based on it can be built using python. Without further ado, let’s hop into the article.
Aroon Indicator
Founded by Tushar Chande in 1995, the Aroon indicator is a momentum oscillator that is specifically designed to track a market’s trend and how strong the trend is. This indicator is widely used by traders to identify a new trend in the market and make potential entry and exit points accordingly. Being an oscillator, the values of the Aroon indicator bound between 0 to 100.
The Aroon indicator is composed of two components: The Aroon up line and the Aroon down line. The Aroon up line measures the strength of the uptrend in a market and similarly, the Aroon down line measures the strength of the downtrend in a market. The traditional setting for the Aroon indicator is either 14 for short-term or 25 for long-term as the lookback period. In this article, we will be using 25 as the setting since we will be dealing with a year and a half of data. The formula to calculate these two lines with 25 as the lookback period are as follows:
AROON UP = [ 25 - PERIODS SINCE 25 PERIOD HIGH ] / 25 * [ 100 ]
AROON DOWN = [ 25 - PERIODS SINCE 25 PERIOD LOW ] / 25 * [ 100 ]
The Aroon up line is calculated by first determining how long has it been since the stock has reached a new high over a 25-day timeframe, then, subtract and divide this value by 25 and finally multiply it by 100. This applies to calculating the Aroon down line too but here we are determining the number of days since a new low has occurred instead of observing new highs.
The main concept of the Aroon indicator is that the market tends to attain more new highs during a strong uptrend, and similarly, the market is bound to reach more new lows during a sturdy downtrend. With that being said, let’s explore how the Aroon indicator can be used to build a trading strategy.
To my knowledge, the Aroon Indicator can be used in two ways to build a trading strategy. First is the crossover trading strategy. This strategy reveals a buy signal if the Aroon up line crosses from below to above the Aroon down line, similarly, a sell signal is revealed if the Aroon up line moves from above to below the Aroon down line. The crossover strategy can be represented as follows:
IF P.UP LINE < P.DOWN LINE AND C.UP LINE > C.DOWN LINE --> BUY
IF P.UP LINE > P.DOWN LINE AND C.UP LINE < C.DOWN LINE --> SELL
The second strategy is constructing a higher and lower threshold which represents a buy signal if the Aroon up line has a reading of and above 70 and parallelly, the Aroon down line has a reading of and below 30. Likewise, when the Aroon up line has a reading of or below 30 and the reading of Aroon down line is observed to be at or above 70, a sell signal is revealed. This strategy can be represented as follows:
IF AROON UP LINE => 70 AND AROON DOWN LINE <= 30 --> BUY SIGNAL
IF AROON UP LINE <= 30 AND AROON DOWN LINE >= 70 --> SELL SIGNAL
Both the trading strategies are highly effective and can be used to trade any stocks. In this article, we are going to implement the second trading strategy of constructing thresholds just to make things simple. Now that we have an idea of what the Aroon indicator is all about, its calculation and usage. Let’s proceed to the programming part where we will build the second Aroon indicator strategy from scratch in python and backtest it on Tesla. Before moving on, a note on disclaimer: This article’s sole purpose is to educate people and must be considered as an information piece but not as investment advice or so.
Implementation in Python
The coding part is classified into various steps as follows:
1. Importing Packages
2. Extracting Stock Data from Twelve Data
3. Extracting the Aroon Indicator values
4. Aroon Indicator Plot
5. Creating the Trading Strategy
6. Plotting the Trading Lists
7. Creating our Position
8. Backtesting
9. SPY ETF Comparison
We will be following the order mentioned in the above list and buckle up your seat belts to follow every upcoming coding part.
Step-1: Importing Packages
Importing the required packages into the python environment is a non-skippable step. The primary packages are going to be Pandas to work with data, NumPy to work with arrays and for complex functions, Matplotlib for plotting purposes, and Requests to make API calls. The secondary packages are going to be Math for mathematical functions and Termcolor for font customization (optional).
Python Implementation:
import pandas as pd
import numpy as np
import requests
import matplotlib.pyplot as plt
from math import floor
from termcolor import colored as cl
plt.style.use('fivethirtyeight')
plt.rcParams['figure.figsize'] = (20, 10)
Now that we have imported all the required packages into our python. Let’s pull the historical data of Tesla with Twelve Data’s API endpoint.
Step-2: Extracting data from Twelve Data
In this step, we are going to pull the historical stock data of Tesla using an API endpoint provided by twelvedata.com. Before that, a note on twelvedata.com: Twelve Data is one of the leading market data providers having an enormous amount of API endpoints for all types of market data. It is very easy to interact with the APIs provided by Twelve Data and has one of the best documentation ever. Also, ensure that you have an account on twelvedata.com, only then, you will be able to access your API key (vital element to extract data with an API).
Python Implementation:
def get_historical_data(symbol, start_date):
api_key = 'YOUR API KEY'
api_url = f'https://api.twelvedata.com/time_series?symbol={symbol}&interval=1day&outputsize=5000&apikey={api_key}'
raw_df = requests.get(api_url).json()
df = pd.DataFrame(raw_df['values']).iloc[::-1].set_index('datetime').astype(float)
df = df[df.index >= start_date]
df.index = pd.to_datetime(df.index)
return df
tsla = get_historical_data('TSLA', '2020-01-01')
tsla
Output:
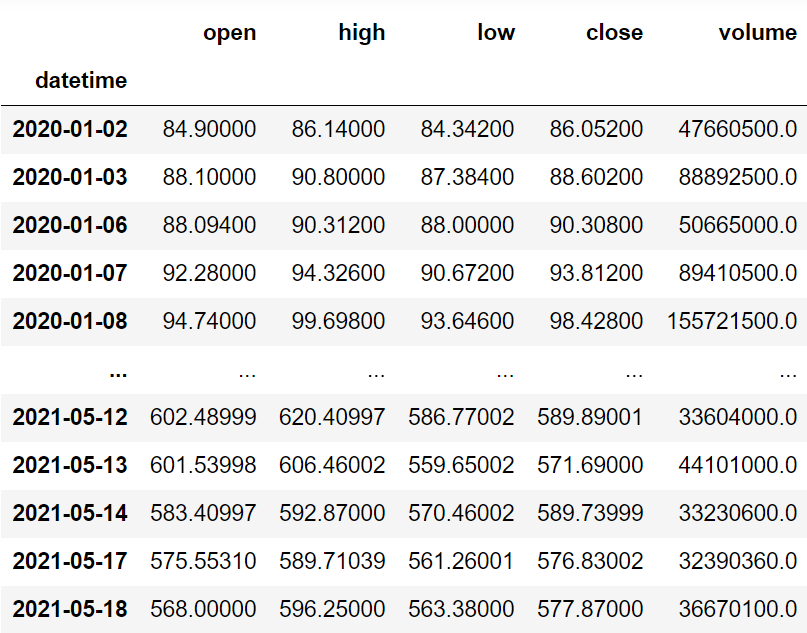
Code Explanation: The first thing we did is to define a function named ‘get_historical_data’ that takes the stock’s symbol (‘symbol’) and the starting date of the historical data (‘start_date’) as parameters. Inside the function, we are defining the API key and the URL and stored them into their respective variable. Next, we are extracting the historical data in JSON format using the ‘get’ function and stored it into the ‘raw_df’ variable. After doing some processes to clean and format the raw JSON data, we are returning it in the form of a clean Pandas dataframe. Finally, we are calling the created function to pull the historic data of Tesla from the starting of 2020 and stored it into the ‘tsla’ variable.
Step-3: Extracting the Aroon Indicator values
In this step, we are going to pull the Aroon indicator values of Tesla with the help of an API endpoint provided by Twelve Data. This step is almost similar to what we did in the previous step.
Python Implementation:
def get_aroon(symbol, lookback, start_date):
api_key = 'YOUR API KEY'
api_url = f'https://api.twelvedata.com/aroon?symbol={symbol}&interval=1day&time_period={lookback}&outputsize=5000&apikey={api_key}'
raw_df = requests.get(api_url).json()
df = pd.DataFrame(raw_df['values']).iloc[::-1].set_index('datetime').astype(float)
df = df[df.index >= start_date]
df.index = pd.to_datetime(df.index)
aroon_up = df['aroon_up']
aroon_down = df['aroon_down']
return aroon_up, aroon_down
tsla['aroon_up'], tsla['aroon_down'] = get_aroon('TSLA', 25, '2020-01-01')
tsla.tail()
Output:

Code Explanation: Firstly, we are defining a function named ‘get_aroon’ which takes the stock’s symbol (‘symbol’), the lookback period for the indicator (‘lookback’), and the starting date of the data (‘start_date’) as parameters. Inside the function, we are first assigning two variables named ‘api_key’ and ‘url’ to store the API key and the API URL respectively. Using the ‘get’ function provided by the Requests package, we are calling the API and stored the response into the ‘raw’ variable. After doing some data manipulations, we are returning both the Aroon up line and down line values. Finally, we are calling the function to extract the Aroon indicator values of Tesla.
Step-4: Aroon Indicator Plot
In this step, we are going to plot the extracted Aroon indicator values of Tesla to make more sense out of it. The main aim of this part is not on the coding section but instead to observe the plot to gain a solid understanding of the Aroon indicator.
Python Implementation:
ax1 = plt.subplot2grid((11,1), (0,0), rowspan = 5, colspan = 1)
ax2 = plt.subplot2grid((11,1), (6,0), rowspan = 4, colspan = 1)
ax1.plot(tsla['close'], linewidth = 2.5, color = '#2196f3')
ax1.set_title('TSLA CLOSE PRICES')
ax2.plot(tsla['aroon_up'], color = '#26a69a', linewidth = 2, label = 'AROON UP')
ax2.plot(tsla['aroon_down'], color = '#ef5350', linewidth = 2, label = 'AROON DOWN')
ax2.legend()
ax2.set_title('TSLA AROON 25')
plt.show()
Output:
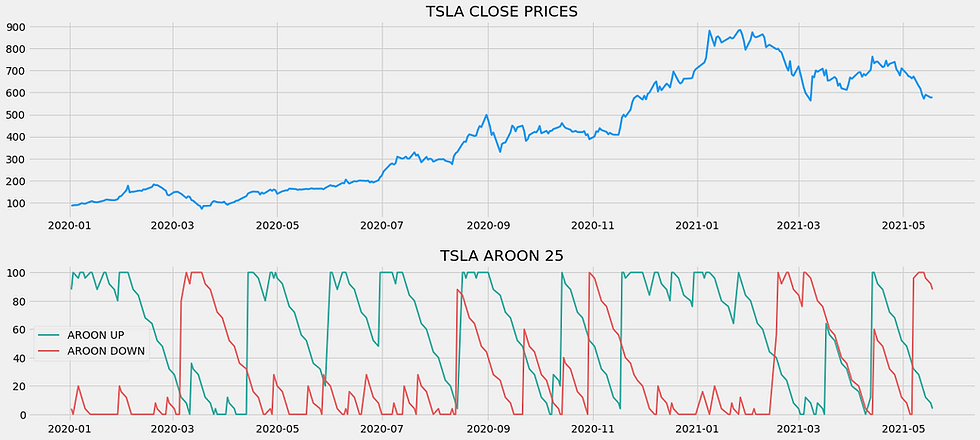
The above chart is divided into two panels: the above panel comprising the plot of the closing prices of Tesla and the lower panel with both the Aroon up and down lines. I mentioned that the Aroon indicator is not only useful for identifying trends but also for identifying ranging markets. To identify ranging market periods, we have to carefully observe the up and down lines. Whenever the space between the two lines is less, then the market is said to be ranging. Similarly, trending markets are observed when the space between the two lines is wider.
You could also see that whenever the market seems to be rising in a strong uptrend, the readings of the Aroon up line also rises, parallelly, the readings of the Aroon down line decreases. Likewise, during a period of a strong downtrend, the readings of the Aroon down line crosses above the Aroon up line. From this, we could say that both the lines are inversely proportional to each other.
Sometimes, it is observable that the Aroon up line stays at 100 for a while. This represents that market is in a very strong uptrend creating new highs. This applies to the Aroon down line too. The market is said to be highly bearish creating new lows when the Aroon down line stays at 100 for a while. This feature of the Aroon indicator to identify very strong uptrends and downtrends comes in handy for traders in the real-world market.
Step-5: Creating the trading strategy
In this step, we are going to implement the discussed Aroon Indicator trading strategy in python.
Python Implementation:
def implement_aroon_strategy(prices, up, down):
buy_price = []
sell_price = []
aroon_signal = []
signal = 0
for i in range(len(prices)):
if up[i] >= 70 and down[i] <= 30:
if signal != 1:
buy_price.append(prices[i])
sell_price.append(np.nan)
signal = 1
aroon_signal.append(signal)
else:
buy_price.append(np.nan)
sell_price.append(np.nan)
aroon_signal.append(0)
elif up[i] <= 30 and down[i] >= 70:
if signal != -1:
buy_price.append(np.nan)
sell_price.append(prices[i])
signal = -1
aroon_signal.append(signal)
else:
buy_price.append(np.nan)
sell_price.append(np.nan)
aroon_signal.append(0)
else:
buy_price.append(np.nan)
sell_price.append(np.nan)
aroon_signal.append(0)
return buy_price, sell_price, aroon_signal
buy_price, sell_price, aroon_signal = implement_aroon_strategy(tsla['close'], tsla['aroon_up'], tsla['aroon_down'])
Code Explanation: First, we are defining a function named ‘implement_aroon_strategy’ which takes the stock prices (‘price), and the lines of the Aroon indicator (‘up’, ‘down’) as parameters.
Inside the function, we are creating three empty lists (buy_price, sell_price, and aroon_signal) in which the values will be appended while creating the trading strategy.
After that, we are implementing the trading strategy through a for-loop. Inside the for-loop, we are passing certain conditions, and if the conditions are satisfied, the respective values will be appended to the empty lists. If the condition to buy the stock gets satisfied, the buying price will be appended to the ‘buy_price’ list, and the signal value will be appended as 1 representing to buy the stock. Similarly, if the condition to sell the stock gets satisfied, the selling price will be appended to the ‘sell_price’ list, and the signal value will be appended as -1 representing to sell the stock.
Finally, we are returning the lists appended with values. Then, we are calling the created function and stored the values into their respective variables. The list doesn’t make any sense unless we plot the values. So, let’s plot the values of the created trading lists.
Step-6: Plotting the trading signals
In this step, we are going to plot the created trading lists to make sense out of them.
Python Implementation:
ax1 = plt.subplot2grid((11,1), (0,0), rowspan = 5, colspan = 1)
ax2 = plt.subplot2grid((11,1), (6,0), rowspan = 4, colspan = 1)
ax1.plot(tsla['close'], linewidth = 2.5, color = '#2196f3')
ax1.plot(tsla.index, buy_price, marker = '^', color = '#26a69a', markersize = 12)
ax1.plot(tsla.index, sell_price, marker = 'v', color = '#ef5350', markersize = 12)
ax1.set_title('TSLA CLOSE PRICES')
ax2.plot(tsla['aroon_up'], color = '#26a69a', linewidth = 2, label = 'AROON UP')
ax2.plot(tsla['aroon_down'], color = '#ef5350', linewidth = 2, label = 'AROON DOWN')
ax2.legend()
ax2.set_title('TSLA AROON 25')
plt.show()
Output:
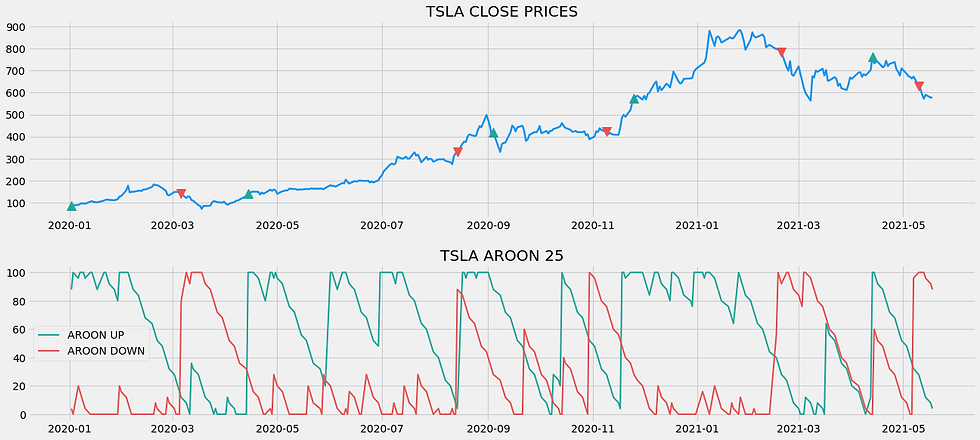
Code Explanation: We are plotting the Aroon indicator components along with the buy and sell signals generated by the trading strategy. We can observe that whenever the Aroon up line has a reading of and above 70 and the Aroon down line has a reading of and below 30, a green-colored buy signal is plotted in the chart. Similarly, whenever the Aroon up line has a reading of and below 30 and the Aroon down line has a reading of and above 70, a red-colored sell signal is plotted in the chart.
Step-7: Creating our Position
In this step, we are going to create a list that indicates 1 if we hold the stock or 0 if we don’t own or hold the stock.
Python Implementation:
position = []
for i in range(len(aroon_signal)):
if aroon_signal[i] > 1:
position.append(0)
else:
position.append(1)
for i in range(len(tsla['close'])):
if aroon_signal[i] == 1:
position[i] = 1
elif aroon_signal[i] == -1:
position[i] = 0
else:
position[i] = position[i-1]
aroon_up = tsla['aroon_up']
aroon_down = tsla['aroon_down']
close_price = tsla['close']
aroon_signal = pd.DataFrame(aroon_signal).rename(columns = {0:'aroon_signal'}).set_index(tsla.index)
position = pd.DataFrame(position).rename(columns = {0:'aroon_position'}).set_index(tsla.index)
frames = [close_price, aroon_up, aroon_down, aroon_signal, position]
strategy = pd.concat(frames, join = 'inner', axis = 1)
strategy
Output:
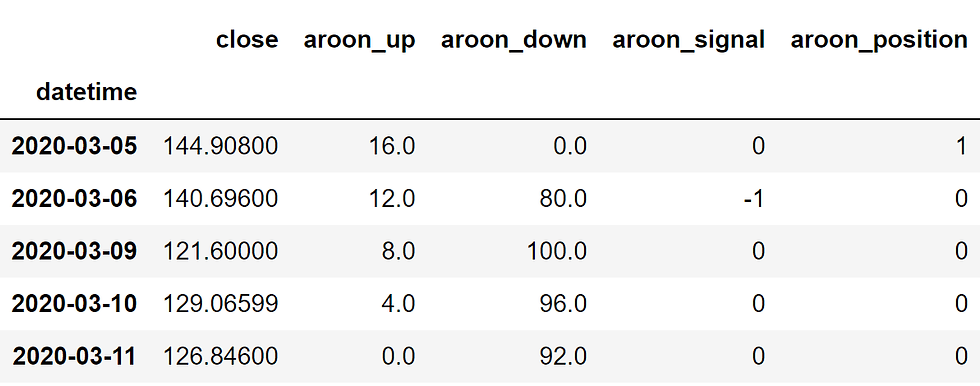
Code Explanation: First, we are creating an empty list named ‘position’. We are passing two for-loops, one is to generate values for the ‘position’ list to just match the length of the ‘signal’ list. The other for-loop is the one we are using to generate actual position values. Inside the second for-loop, we are iterating over the values of the ‘signal’ list, and the values of the ‘position’ list get appended concerning which condition gets satisfied. The value of the position remains 1 if we hold the stock or remains 0 if we sold or don’t own the stock. Finally, we are doing some data manipulations to combine all the created lists into one dataframe.
From the output being shown, we can see that in the first row our position in the stock has remained 1 (since there isn’t any change in the Aroon indicator signal) but our position suddenly turned to -1 as we sold the stock when the Aroon indicator trading signal represents a sell signal (-1). Our position will remain 0 until some changes in the trading signal occur. Now it’s time to do implement some backtesting process!
Step-8: Backtesting
Before moving on, it is essential to know what backtesting is. Backtesting is the process of seeing how well our trading strategy has performed on the given stock data. In our case, we are going to implement a backtesting process for our Aroon indicator trading strategy over the Tesla stock data.
Python Implementation:
tsla_ret = pd.DataFrame(np.diff(tsla['close'])).rename(columns = {0:'returns'})
aroon_strategy_ret = []
for i in range(len(tsla_ret)):
returns = tsla_ret['returns'][i]*strategy['aroon_position'][i]
aroon_strategy_ret.append(returns)
aroon_strategy_ret_df = pd.DataFrame(aroon_strategy_ret).rename(columns = {0:'aroon_returns'})
investment_value = 100000
number_of_stocks = floor(investment_value/tsla['close'][-1])
aroon_investment_ret = []
for i in range(len(aroon_strategy_ret_df['aroon_returns'])):
returns = number_of_stocks*aroon_strategy_ret_df['aroon_returns'][i]
aroon_investment_ret.append(returns)
aroon_investment_ret_df = pd.DataFrame(aroon_investment_ret).rename(columns = {0:'investment_returns'})
total_investment_ret = round(sum(aroon_investment_ret_df['investment_returns']), 2)
profit_percentage = floor((total_investment_ret/investment_value)*100)
print(cl('Profit gained from the Aroon strategy by investing $100k in TSLA : {}'.format(total_investment_ret), attrs = ['bold']))
print(cl('Profit percentage of the Aroon strategy : {}%'.format(profit_percentage), attrs = ['bold']))
Output:
Profit gained from the Aroon strategy by investing $100k in TSLA : 55354.46
Profit percentage of the Aroon strategy : 55%
Code Explanation: First, we are calculating the returns of the Tesla stock using the ‘diff’ function provided by the NumPy package and we have stored it as a dataframe into the ‘tsla_ret’ variable. Next, we are passing a for-loop to iterate over the values of the ‘tsla_ret’ variable to calculate the returns we gained from our Aroon indicator trading strategy, and these returns values are appended to the ‘aroon_strategy_ret’ list. Next, we are converting the ‘aroon_strategy_ret’ list into a dataframe and stored it into the ‘aroon_strategy_ret_df’ variable.
Next comes the backtesting process. We are going to backtest our strategy by investing a hundred thousand USD into our trading strategy. So first, we are storing the amount of investment into the ‘investment_value’ variable. After that, we are calculating the number of Tesla stocks we can buy using the investment amount. You can notice that I’ve used the ‘floor’ function provided by the Math package because, while dividing the investment amount by the closing price of Tesla stock, it spits out an output with decimal numbers. The number of stocks should be an integer but not a decimal number. Using the ‘floor’ function, we can cut out the decimals. Remember that the ‘floor’ function is way more complex than the ‘round’ function. Then, we are passing a for-loop to find the investment returns followed by some data manipulations tasks.
Finally, we are printing the total return we got by investing a hundred thousand into our trading strategy and it is revealed that we have made an approximate profit of fifty-five thousand USD in one year. That’s not bad! Now, let’s compare our returns with SPY ETF (an ETF designed to track the S&P 500 stock market index) returns.
Step-9: SPY ETF Comparison
This step is optional but it is highly recommended as we can get an idea of how well our trading strategy performs against a benchmark (SPY ETF). In this step, we are going to extract the data of the SPY ETF using the ‘get_historical_data’ function we created and compare the returns we get from the SPY ETF with our Aroon indicator strategy returns on Tesla.
Python Implementation:
def get_benchmark(start_date, investment_value):
spy = get_historical_data('SPY', start_date)['close']
benchmark = pd.DataFrame(np.diff(spy)).rename(columns = {0:'benchmark_returns'})
investment_value = investment_value
number_of_stocks = floor(investment_value/spy[-1])
benchmark_investment_ret = []
for i in range(len(benchmark['benchmark_returns'])):
returns = number_of_stocks*benchmark['benchmark_returns'][i]
benchmark_investment_ret.append(returns)
benchmark_investment_ret_df = pd.DataFrame(benchmark_investment_ret).rename(columns = {0:'investment_returns'})
return benchmark_investment_ret_df
benchmark = get_benchmark('2020-01-01', 100000)
investment_value = 100000
total_benchmark_investment_ret = round(sum(benchmark['investment_returns']), 2)
benchmark_profit_percentage = floor((total_benchmark_investment_ret/investment_value)*100)
print(cl('Benchmark profit by investing $100k : {}'.format(total_benchmark_investment_ret), attrs = ['bold']))
print(cl('Benchmark Profit percentage : {}%'.format(benchmark_profit_percentage), attrs = ['bold']))
print(cl('Aroon Strategy profit is {}% higher than the Benchmark Profit'.format(profit_percentage - benchmark_profit_percentage), attrs = ['bold']))
Output:
Benchmark profit by investing $100k : 21090.3
Benchmark Profit percentage : 21%
Aroon Strategy profit is 34% higher than the Benchmark Profit
Code Explanation: The code used in this step is almost similar to the one used in the previous backtesting step but, instead of investing in Tesla, we are investing in SPY ETF by not implementing any trading strategies. From the output, we can see that our Aroon indicator trading strategy has outperformed the SPY ETF by 34%. That’s great!
Final Thoughts!
After a long process of crushing both the theory and coding part, we have successfully learned what the Aroon indicator is all about and how to build a trading strategy based on it. The Aroon indicator has ever stayed my personal favorite since it helps in getting two jobs done at a time (identify trends, volatility), and it's unique too. Even though we have surpassed the returns of SPY ETF, there are still spaces to improve the work:
Strategy optimization: In this article, we have used one of the most basic trading strategies based on the Aroon indicator and this strategy can be used just for the purpose of understanding or gaining intuitions about the indicator but it’s not possible to expect visible returns in the real-world market. So, I highly recommend you to discover other advanced Aroon-based trading strategies like the breakout strategy, pull back strategy, and so on. Apart from just discovering, backtest the strategy with as many stocks as possible as the results might vary from one to another.
Risk Management: When it comes to either trading or long-term investing, this topic is a must to be considered. As long as you hold a tight grasp of this concept, you hold a strong edge in the market. We didn’t cover this topic as the sole purpose of this article is to just understand and brainstorm about the underlying idea of the Aroon indicator but not to make profits. But, it is highly recommended to study in and out about this subject before entering the crazy real-world market.
With that being said, we have come to the end of the article. Hope you learned something useful from this article. Also, if you forgot to follow any of the coding parts, don’t worry. I’ve provided the full source code at the end of the article.
Full code:
import pandas as pd
import numpy as np
import requests
import matplotlib.pyplot as plt
from math import floor
from termcolor import colored as cl
plt.style.use('fivethirtyeight')
plt.rcParams['figure.figsize'] = (20, 10)
def get_historical_data(symbol, start_date):
api_key = 'ace6a59ff46446659af4e691fab88e22'
api_url = f'https://api.twelvedata.com/time_series?symbol={symbol}&interval=1day&outputsize=5000&apikey={api_key}'
raw_df = requests.get(api_url).json()
df = pd.DataFrame(raw_df['values']).iloc[::-1].set_index('datetime').astype(float)
df = df[df.index >= start_date]
df.index = pd.to_datetime(df.index)
return df
tsla = get_historical_data('TSLA', '2020-01-01')
print(tsla)
def get_aroon(symbol, lookback, start_date):
api_key = 'ace6a59ff46446659af4e691fab88e22'
api_url = f'https://api.twelvedata.com/aroon?symbol={symbol}&interval=1day&time_period={lookback}&outputsize=5000&apikey={api_key}'
raw_df = requests.get(api_url).json()
df = pd.DataFrame(raw_df['values']).iloc[::-1].set_index('datetime').astype(float)
df = df[df.index >= start_date]
df.index = pd.to_datetime(df.index)
aroon_up = df['aroon_up']
aroon_down = df['aroon_down']
return aroon_up, aroon_down
tsla['aroon_up'], tsla['aroon_down'] = get_aroon('TSLA', 25, '2020-01-01')
print(tsla.tail())
ax1 = plt.subplot2grid((11,1), (0,0), rowspan = 5, colspan = 1)
ax2 = plt.subplot2grid((11,1), (6,0), rowspan = 4, colspan = 1)
ax1.plot(tsla['close'], linewidth = 2.5, color = '#2196f3')
ax1.set_title('TSLA CLOSE PRICES')
ax2.plot(tsla['aroon_up'], color = '#26a69a', linewidth = 2, label = 'AROON UP')
ax2.plot(tsla['aroon_down'], color = '#ef5350', linewidth = 2, label = 'AROON DOWN')
ax2.legend()
ax2.set_title('TSLA AROON 25')
plt.show()
def implement_aroon_strategy(prices, up, down):
buy_price = []
sell_price = []
aroon_signal = []
signal = 0
for i in range(len(prices)):
if up[i] >= 70 and down[i] <= 30:
if signal != 1:
buy_price.append(prices[i])
sell_price.append(np.nan)
signal = 1
aroon_signal.append(signal)
else:
buy_price.append(np.nan)
sell_price.append(np.nan)
aroon_signal.append(0)
elif up[i] <= 30 and down[i] >= 70:
if signal != -1:
buy_price.append(np.nan)
sell_price.append(prices[i])
signal = -1
aroon_signal.append(signal)
else:
buy_price.append(np.nan)
sell_price.append(np.nan)
aroon_signal.append(0)
else:
buy_price.append(np.nan)
sell_price.append(np.nan)
aroon_signal.append(0)
return buy_price, sell_price, aroon_signal
buy_price, sell_price, aroon_signal = implement_aroon_strategy(tsla['close'], tsla['aroon_up'], tsla['aroon_down'])
ax1 = plt.subplot2grid((11,1), (0,0), rowspan = 5, colspan = 1)
ax2 = plt.subplot2grid((11,1), (6,0), rowspan = 4, colspan = 1)
ax1.plot(tsla['close'], linewidth = 2.5, color = '#2196f3')
ax1.plot(tsla.index, buy_price, marker = '^', color = '#26a69a', markersize = 12)
ax1.plot(tsla.index, sell_price, marker = 'v', color = '#ef5350', markersize = 12)
ax1.set_title('TSLA CLOSE PRICES')
ax2.plot(tsla['aroon_up'], color = '#26a69a', linewidth = 2, label = 'AROON UP')
ax2.plot(tsla['aroon_down'], color = '#ef5350', linewidth = 2, label = 'AROON DOWN')
ax2.legend()
ax2.set_title('TSLA AROON 25')
plt.show()
position = []
for i in range(len(aroon_signal)):
if aroon_signal[i] > 1:
position.append(0)
else:
position.append(1)
for i in range(len(tsla['close'])):
if aroon_signal[i] == 1:
position[i] = 1
elif aroon_signal[i] == -1:
position[i] = 0
else:
position[i] = position[i-1]
aroon_up = tsla['aroon_up']
aroon_down = tsla['aroon_down']
close_price = tsla['close']
aroon_signal = pd.DataFrame(aroon_signal).rename(columns = {0:'aroon_signal'}).set_index(tsla.index)
position = pd.DataFrame(position).rename(columns = {0:'aroon_position'}).set_index(tsla.index)
frames = [close_price, aroon_up, aroon_down, aroon_signal, position]
strategy = pd.concat(frames, join = 'inner', axis = 1)
strategy.head()
print(strategy[43:48])
tsla_ret = pd.DataFrame(np.diff(tsla['close'])).rename(columns = {0:'returns'})
aroon_strategy_ret = []
for i in range(len(tsla_ret)):
returns = tsla_ret['returns'][i]*strategy['aroon_position'][i]
aroon_strategy_ret.append(returns)
aroon_strategy_ret_df = pd.DataFrame(aroon_strategy_ret).rename(columns = {0:'aroon_returns'})
investment_value = 100000
number_of_stocks = floor(investment_value/tsla['close'][-1])
aroon_investment_ret = []
for i in range(len(aroon_strategy_ret_df['aroon_returns'])):
returns = number_of_stocks*aroon_strategy_ret_df['aroon_returns'][i]
aroon_investment_ret.append(returns)
aroon_investment_ret_df = pd.DataFrame(aroon_investment_ret).rename(columns = {0:'investment_returns'})
total_investment_ret = round(sum(aroon_investment_ret_df['investment_returns']), 2)
profit_percentage = floor((total_investment_ret/investment_value)*100)
print(cl('Profit gained from the Aroon strategy by investing $100k in TSLA : {}'.format(total_investment_ret), attrs = ['bold']))
print(cl('Profit percentage of the Aroon strategy : {}%'.format(profit_percentage), attrs = ['bold']))
def get_benchmark(start_date, investment_value):
spy = get_historical_data('SPY', start_date)['close']
benchmark = pd.DataFrame(np.diff(spy)).rename(columns = {0:'benchmark_returns'})
investment_value = investment_value
number_of_stocks = floor(investment_value/spy[-1])
benchmark_investment_ret = []
for i in range(len(benchmark['benchmark_returns'])):
returns = number_of_stocks*benchmark['benchmark_returns'][i]
benchmark_investment_ret.append(returns)
benchmark_investment_ret_df = pd.DataFrame(benchmark_investment_ret).rename(columns = {0:'investment_returns'})
return benchmark_investment_ret_df
benchmark = get_benchmark('2020-01-01', 100000)
investment_value = 100000
total_benchmark_investment_ret = round(sum(benchmark['investment_returns']), 2)
benchmark_profit_percentage = floor((total_benchmark_investment_ret/investment_value)*100)
print(cl('Benchmark profit by investing $100k : {}'.format(total_benchmark_investment_ret), attrs = ['bold']))
print(cl('Benchmark Profit percentage : {}%'.format(benchmark_profit_percentage), attrs = ['bold']))
print(cl('Aroon Strategy profit is {}% higher than the Benchmark Profit'.format(profit_percentage - benchmark_profit_percentage), attrs = ['bold']))
Good one Nikhil
Useful one. Good Nikhil